FileUpload Control Validation
With FileUpload control you can receive files from your web application users. Often, you don't want to receive all file types, but only specific extensions (e.g. images only) depending of your application requirements. Unfortunately, FileUpload control still have not some Filter property like Open and Save File dialogs in .NET Windows Forms to limit file types. Because of that, you need to write some additional code to be sure that user will upload regular file type.
Let say you have two web controls on web form, on FileUpload Control to select a file and one button control, like in image bellow:
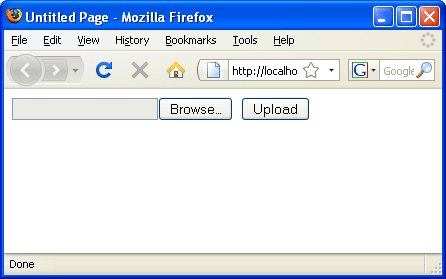
Image 1: FileUpload and Button on web form
Because in ASP.NET we have client and server side, validation could be done on client and on server. You need to have server side validation because of security reasons, but you also need client side validation because it is faster and more user friendly. Let see how to implement both types, for example our web application will allow only upload of .xls or .xml files.
FileUpload Control client side validation
You can use CustomValidator to implement FileUpload validation on client side. Possible implementation could be with code like this:
<asp:FileUpload
ID="fuData"
runat="server"
/>
<asp:Button
ID="btnUpload"
runat="server"
Text="Upload"
/>
<br
/>
<asp:CustomValidator
ID="CustomValidator1"
runat="server"
ClientValidationFunction="ValidateFileUpload"
ErrorMessage="Please
select valid .xls or .xml file"></asp:CustomValidator>
<script
language="javascript"
type="text/javascript">
function
ValidateFileUpload(Source, args)
{
var fuData = document.getElementById('<%=
fuData.ClientID %>');
var FileUploadPath = fuData.value;
if(FileUploadPath =='')
{
// There is no file selected
args.IsValid =
false;
}
else
{
var Extension =
FileUploadPath.substring(FileUploadPath.lastIndexOf('.')
+ 1).toLowerCase();
if (Extension ==
"xls" || Extension == "xml")
{
args.IsValid =
true; // Valid file
type
}
else
{
args.IsValid =
false; // Not valid
file type
}
}
}
</script>
If visitor tries to upload wrong file type or FileUpload have empty value, CustomValidator will return an error message, like in image bellow:
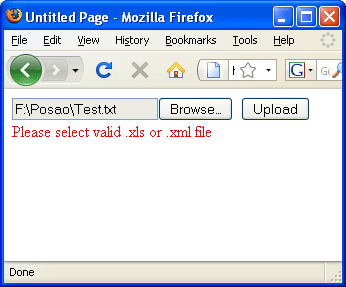
Image 2: Error message is shown
FileUpload Control server side validation
Because of security reasons, you need to validate FileUpload control on server side too. You can use the same idea like for client side validation. In this case, just use OnServerValidate event, like in code bellow:
[ C# ]
protected
void CustomValidator1_ServerValidate(object
source, ServerValidateEventArgs args)
{
// Get file name
string UploadFileName =
fuData.PostedFile.FileName;
if(UploadFileName ==
"")
{
// There is no file selected
args.IsValid =
false;
}
else
{
string Extension =
UploadFileName.Substring(UploadFileName.LastIndexOf('.')
+ 1).toLower();
if (Extension ==
"xls" || Extension == "xml")
{
args.IsValid
= true; // Valid file
type
}
else
{
args.IsValid
= false; // Not valid
file type
}
}
}
[ VB.NET ]
Protected
Sub CustomValidator1_ServerValidate(ByVal
source As Object,
ByVal args As
System.Web.UI.WebControls.ServerValidateEventArgs)
Handles CustomValidator1.ServerValidate
' Get file name
Dim UploadFileName As
String = fuData.PostedFile.FileName
If UploadFileName =
"" Then
' There is no file selected
args.IsValid =
False
Else
Dim Extension As
String =
UploadFileName.Substring(UploadFileName.LastIndexOf(".")
+ 1).ToLower()
If Extension = "xls"
Or Extension = "xml"
Then
args.IsValid =
True ' Valid file type
Else
args.IsValid =
False ' Not valid
file type
End If
End If
End
Sub
Now is your FileUpload Control protected on client and server side. I hope you found this tutorial helpful.
Happy programming!
Related articles:
1. Solving "Image Not Found" Problem In GridView