How To Create Thumbnail From Larger Image?
If you are creating an image gallery application, you need to show smaller images (thumbnails) so visitors could browse gallery easily. Also, if users of your web application have an option to upload images, you probably need to check image size and shrink it if image is too large and can't fit in page design.
This function will create thumbnail on disk from existing larger image:
[ C# ]
using System;
// Include these namespaces
using System.Drawing;
using System.Drawing.Drawing2D;
...
void CreateThumbnail(int ThumbnailMax, string OriginalImagePath, string ThumbnailImagePath)
{
// Loads original image from file
Image imgOriginal = Image.FromFile(OriginalImagePath);
// Finds height and width of original image
float OriginalHeight = imgOriginal.Height;
float OriginalWidth = imgOriginal.Width;
// Finds height and width of resized image
int ThumbnailWidth;
int ThumbnailHeight;
if (OriginalHeight > OriginalWidth)
{
ThumbnailHeight = ThumbnailMax;
ThumbnailWidth = (int)((OriginalWidth / OriginalHeight) * (float)ThumbnailMax);
}
else
{
ThumbnailWidth = ThumbnailMax;
ThumbnailHeight = (int)((OriginalHeight / OriginalWidth) * (float)ThumbnailMax);
}
// Create new bitmap that will be used for thumbnail
Bitmap ThumbnailBitmap = new Bitmap(ThumbnailWidth, ThumbnailHeight);
Graphics ResizedImage = Graphics.FromImage(ThumbnailBitmap);
// Resized image will have best possible quality
ResizedImage.InterpolationMode = InterpolationMode.HighQualityBicubic;
ResizedImage.CompositingQuality = CompositingQuality.HighQuality;
ResizedImage.SmoothingMode = SmoothingMode.HighQuality;
// Draw resized image
ResizedImage.DrawImage(imgOriginal, 0, 0, ThumbnailWidth, ThumbnailHeight);
// Save thumbnail to file
ThumbnailBitmap.Save(ThumbnailImagePath);
}
[ VB.NET ]
Imports System
' Include these namespaces
Imports System.Drawing
Imports System.Drawing.Drawing2D
...
Sub CreateThumbnail(ByVal ThumbnailMax As Integer, ByVal OriginalImagePath As String, ByVal ThumbnailImagePath As String)
' Loads original image from file
Dim imgOriginal As Image = Image.FromFile(OriginalImagePath)
' Finds height and width of original image
Dim OriginalHeight As Single = imgOriginal.Height
Dim OriginalWidth As Single = imgOriginal.Width
' Finds height and width of resized image
Dim ThumbnailWidth As Integer
Dim ThumbnailHeight As Integer
If OriginalHeight > OriginalWidth Then
ThumbnailHeight = ThumbnailMax
ThumbnailWidth = (OriginalWidth / OriginalHeight) * ThumbnailMax
Else
ThumbnailWidth = ThumbnailMax
ThumbnailHeight = (OriginalHeight / OriginalWidth) * ThumbnailMax
End If
' Create new bitmap that will be used for thumbnail
Dim ThumbnailBitmap As Bitmap = New Bitmap(ThumbnailWidth, ThumbnailHeight)
Dim ResizedImage As Graphics = Graphics.FromImage(ThumbnailBitmap)
' Resized image will have best possible quality
ResizedImage.InterpolationMode = InterpolationMode.HighQualityBicubic
ResizedImage.CompositingQuality = CompositingQuality.HighQuality
ResizedImage.SmoothingMode = SmoothingMode.HighQuality
' Draw resized image
ResizedImage.DrawImage(imgOriginal, 0, 0, ThumbnailWidth, ThumbnailHeight)
' Save thumbnail to file
ThumbnailBitmap.Save(ThumbnailImagePath)
End Sub
CreateThumbnail function has three parameters:
ThumnailMax - integer, max size of the thumbnail
OriginalImagePath - full path to original image
ThumbnailImagePath - full path where we want to store resized image
For example, let say you want to resize an image named Autumn.jpg, max dimension should be 120 pixels and thumbnail named ThumbAutumn.jpg. Code to call CreateThumbnail function would look like this:
[ C# ]
CreateThumbnail(120, Server.MapPath("~/Autumn.jpg"), Server.MapPath("~/ThumbAutumn.jpg");
[ VB.NET ]
CreateThumbnail(120, Server.MapPath("~/Autumn.jpg"), Server.MapPath("~/ThumbAutumn.jpg")
After execution of code, original and resized image will look like this:
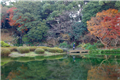
Resized image (thumbnail)
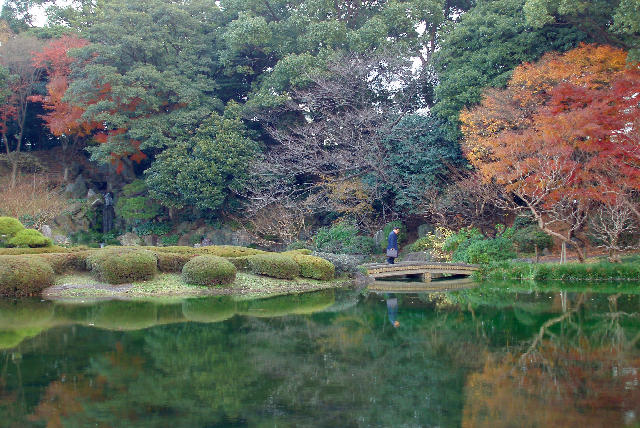
Original image
As you see, this example doesn't use simple Image.GetThumbnailImage method. The code would be shorter if this method is used, but images created with GetThumbnailImage method don't look nice. To get beautiful resized images copy/paste this function to your project and use it where needed. Happy coding!
Related articles:
1. Difference Between Image Class And Bitmap Class?
2. How To Resize All Images In Folder