Solving "Image Not Found" Problem In GridView
With GridView control you have an option to show images by using ImageField column type or TemplateField. By using images your web application could look richer and more professional.
One of the common implementations of GridView with few text columns and images in one column is with code like this:
<asp:GridView
ID="GridView1"
runat="server"
AutoGenerateColumns="False">
<Columns>
<asp:BoundField
DataField="PositionID"
/>
<asp:TemplateField>
<ItemTemplate>
<a
href='<%#
Eval("URL") %>'><h2><%#
Eval("CompanyName")
%></h2></a><br
/>
<%#
Eval("CompanyDesription")
%>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField>
<ItemTemplate>
<a
href='<%#
Eval("URL") %>'>
<asp:Image
BorderStyle="None"
ID="imgLogo"
runat="server"
ImageUrl='~/Images/<%#
Eval("ImageFileName") %>'
/></a>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
But, there is a common problem, what if image file not exists because of some reason? What would happen then?
Well, in that case your web application will not be richer and certainly not look professional. If some image in GridView not exists, ugly red X will be shown, for code snippet above it could produce output like this:
Most useful services for successful ASP.NET developer |
1 |
You can learn any programming language fast, but most important part of anyone success is developing a right personality. Nothing can replace your attitude and because that this item is first on list. Every single dollar you invest in yourself will return in thousands and thousands of others. |
 |
2 |
Carbonite
Carbonite is online backup service, offers unlimited backup space for very low price.Will your business survive a PC Disaster? Start a free trial. |
 |
3 |
GoDaddy
GoDaddy offers domain registrations, ASP.NET web hosting SSL certificates, ecommerce solutions etc., to support your web application in every step. |
 |
4 |
Live Support
If you have some product or service, you can increase sales if you offer fast customer support. Faster service is better service. Install live support to turn your visitors to your customers. |
 |
5 |
NetReactor
NetReactor is very affordable and high quality .NET obfuscator and license maker. |
 |
Case 1: Very useful list, but a little ugly with image not found in 4. row
This is not nice and will not improve your reputation. Fortunately, solution is not complex, you need to create one default image or just white 1x1 pixel image if it is better for your case, and show default image if real image not found. Since case above is about favorite ASP.NET hosting providers, I will place coherent default image like this:
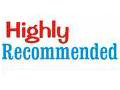 My default image
To show default image only when image from data source is not found you need to use RowDataBound event of GridView control, with code like this:
[ C# ]
// We
need these namespaces
using
System.Web.UI.WebControls;
using
System.IO;
...
///
<summary>
///
Check if current image exists and correct ImageUrl
///
property if image not found
///
</summary>
///
<param name="sender"></param>
///
<param name="e"></param>
protected
void GridView1_RowDataBound(object
sender, GridViewRowEventArgs e)
{
if
(e.Row.RowType == DataControlRowType.DataRow)
{
Image
CurrentImage = (Image)e.Row.FindControl("imgLogo");
if
(!File.Exists(Server.MapPath(CurrentImage.ImageUrl)))
{
// if image not exists, use default image
CurrentImage.ImageUrl
= "~/Images/DefaultImage.gif";
}
}
}
[ VB.NET ]
' We
need this namespaces
Imports
System.Web.UI.WebControls
Imports
System.IO
...
'''
<summary>
'''
Check if current image exists and correct ImageUrl
'''
property if image not found
'''
</summary>
'''
<param name="sender"></param>
'''
<param name="e"></param>
'''
<remarks></remarks>
Protected
Sub GridView1_RowDataBound(ByVal
sender As Object,
ByVal e As
System.Web.UI.WebControls.GridViewRowEventArgs) Handles
GridView1.RowDataBound
If e.Row.RowType = DataControlRowType.DataRow
Then
Dim CurrentImage As
Image = e.Row.FindControl("imgLogo")
If Not File.Exists(Server.MapPath(CurrentImage.ImageUrl))
Then
' if image not exists, use default image
CurrentImage.ImageUrl
= "~/Images/DefaultImage.gif"
End If
End If
End
Sub
Now, when we run the code, output will be more user friendly. If some image is missing, default image will be displayed in GridView:
Most useful services for successful ASP.NET developer |
1 |
You can learn any programming language fast, but most important part of anyone success is developing a right personality. Nothing can replace your attitude and because that this item is first on list. Every single dollar you invest in yourself will return in thousands and thousands of others. |
 |
2 |
Carbonite
Carbonite is online backup service, offers unlimited backup space for very low price.Will your business survive a PC Disaster? Start a free trial. |
 |
3 |
GoDaddy
GoDaddy offers domain registrations, ASP.NET web hosting SSL certificates, ecommerce solutions etc., to support your web application in every step. |
 |
4 |
Live Support
If you have some product or service, you can increase sales if you offer fast customer support. Faster service is better service. Install live support to turn your visitors to your customers. |
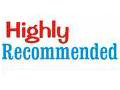 |
5 |
NetReactor
NetReactor is very affordable and high quality .NET obfuscator and license maker. |
 |
I hope this tutorial was useful for you, Happy Programming!
Tutorial toolbar: Tell A Friend | Add to favorites | Feedback |
|