If you have to support n languages, you have to develop n resource files. The naming convention is: The first part of the file name is base name. The second part specifies the culture. This is optional part. If you skip it, the resources in the resource file would be defined for default values.
Example: Your page name is webapp.aspx and you have to include three additional resource files. To include US English, Egyptian Arabic and Israel Hebrew, You have to name these resource files as webapp.aspx.en-US.resx, webapp.aspx.ar-EG.resx, webapp.aspx.he-IL.resx respectively. If you skip the second part of the name, you would be assigned resources on default.
For localizing a page, design a sample page whose controls are to be localized. By sample page, I mean a page whose controls are assigned different language specific values based on the CurrentCulture value of the Current thread, as we have shortly discussed. After designing the page, go to Tools and then select Generate Local Resource.
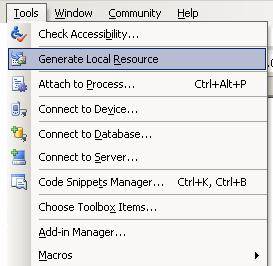
Generating Resource File
A resource file is created which would include the control values for all the controls, you have designed. This file can be seen in the App_LocalResources folder. Its structure is like a hash table. There is Name-Value pair in it. When you open this file, you would find all controls along with their values. An example can be:
LabelResource1.Text = "Your Age" |
To assign language specific values to the controls, copy all the generated resources and paste them giving the file a different name. The naming convention would be same as we discussed. For example, to include the same control in Arabic language, copy all the generated resources, assign the name webapp.aspx.ar.resx and translate values for the Controls. For example:
LabelResource1.Text = "?? ?? ????" |
Here are snapshots of two resource files. The first one contain localizable controls which have been translated in urdu language resource file.
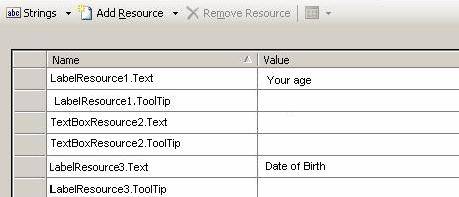
webapp.aspx.en-US.resx
webapp.aspx.ur.resx
Note that all controls which are generated by the Resource generator in Visual studio have to possess a [Localizable] property. In other words, to define a culture specific control, add [localizable] property to it. After generating the resource file, if you see the code for these controls, you would see an implicit localization expression along with code of every control. For example, the code for the Label Ac€~Age' before generating resource file was:
<asp:Label ID= "Age " Text= "Your Age " runat= "server " /> |
And after generating the resource file, you would see something like:
<asp: Label ID= "Age " Text= "Your Age " runat= "server " Meta: resourcekey= "LabelResource1 " /> |
The meta:resourceKey is Localization expression and its value (LabelResource1 in this case) points to the base resource. Similarly, to add other resource files for different languages, just copy all the items from the main resource file and translate the values in the respective resource files.
|