Now let’s edit both the predefined steps and add a completion step that shows the data submitted by the user in the first two steps. The default format of the wizard makes it hard to identify from where the content area begins. Click on the small box that appears at the upper right corner of the control to open the wizard setting menu.
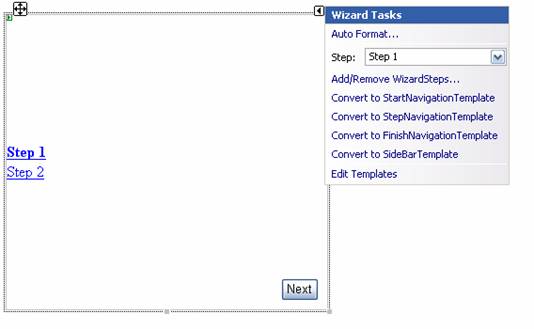
Click on Auto Format to open the Auto Format dialog box:
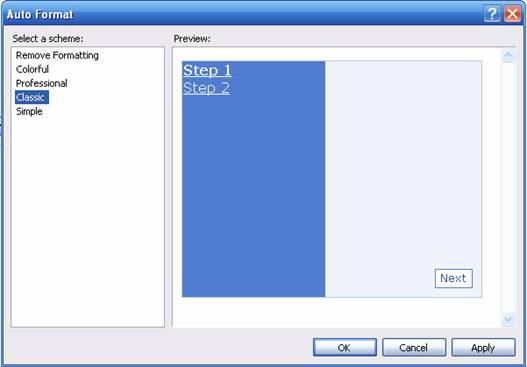
Choose a format that is likeable to you and click OK to apply the format and exit the dialog.
After setting the format, click the underlined Step 1 in the Wizard Control to activate the first step. Click the edit area (the area above the button) to focus on it. You can now edit the display of Step 1.
Suppose we want to get the user's name and e-mail address in this step. After clicking in the edit area, type â€~Name:'. Then drag a TextBox control onto the active area of the wizard, next to the text you just typed. Press enter, type â€~E-Mail Address:' and drag another TextBox onto the active area of the wizard next to the new text.
The end result should be something like this:
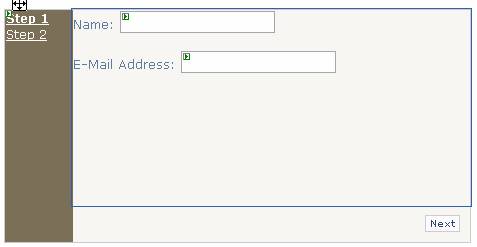
Now, let's move to the second step by clicking on Step 2 in the Wizard Control. Suppose we want the user's opinion or comments in this step. Create a step similar to the following:
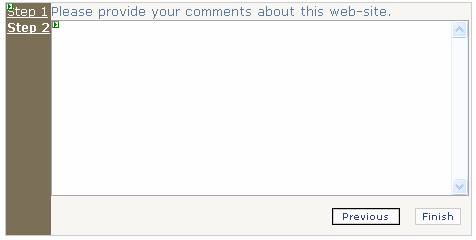
Save the file.
Now we will add a completion step which will display the information the user submitted in the previous two steps.
Right-click the wizard control and click Add/Remove Wizard Steps. The WizardStep Collection Editor appears. From the Add drop-down list on the Add button, select Wizard Step. The Properties area now shows the new step. Set the title of the new step to Completed, and its StepType to Complete. Click on OK button to save and exit.
Now open the Smart Tag – by clicking on the small pictorial box located at the upper right corner of the control. In the Wizard Tasks dialog box, use the Step drop-down list to choose the Finished step. (The name in the drop-down list will be the name you gave the step when you created it, Completed in this example.)
Note that the Sidebar in the Completed Step is not visible. This is to prevent navigation to other steps upon arrival to this step. Drag three labels onto the wizard control. Name the labels lblName, lblMail, and lblComments. Save the file.
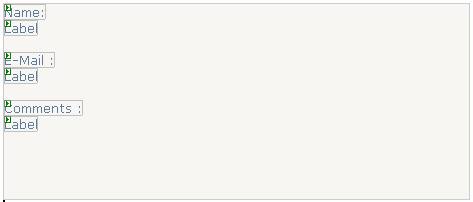
This step will display the data entered by the user in the previous two steps. Using the page's Load event, we can assign the values from the first two steps to the labels in the completion step.
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load lblName.Text = txtName.Text lblMail.Text = txtMail.Text lblComments.Text = txtComments.Text End Sub
Finally, let's test our control. Press CTRL+F5 to compile and execute the application. Type in the textboxes and proceed to the completion step. (Be sure to set the active step to Step 1 before compilation, or it will set the active step for the wizard control to the final step.)
This is the expected result, (with your information of course)
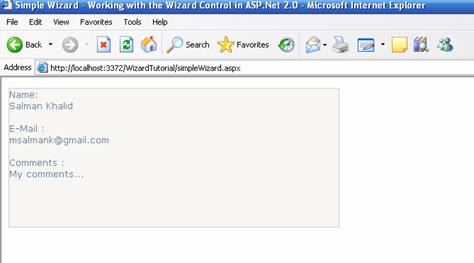
Now let us look at a little advanced scenario. Suppose we want to provide the user with the option to skip entry of comments. To do this, I am going to modify my first step and add a checkbox to the display area, as shown in the figure below:
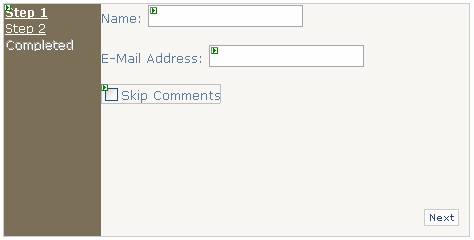
Switch to the code, and create a method to handle the NextButtonClick event of the wizard.
Protected Sub myWizard_NextButtonClick( ByVal sender As Object , ByVal e As System.Web.UI.WebControls.WizardNavigationEventArgs) Handles myWizard.NextButtonClick
Protected Sub myWizard_NextButtonClick(ByVal sender As Object, ByVal e As System.Web.UI.WebControls.WizardNavigationEventArgs) Handles myWizard.NextButtonClick If myWizard.ActiveStepIndex = 0 Then If chkSkip.Checked Then 'Skip the Comments Part myWizard.ActiveStepIndex = 2 lblComments.Visible = False Else myWizard.ActiveStepIndex = 1 End If End If End Sub
|